This article will show you how you can install Zend Framework 2 into your windows OS without composer.phar help.
1. Download latest stable copy of ZF2 from http://framework.zend.com/downloads/latest and unpack, we call it ZF2
2. Download latest stable copy of ZF2 skeleton app from https://github.com/zendframework/ZendSkeletonApplication/ and unpack, we call it ZF2Skeleton
3. Create folder like <ZF2Skeleton folder>/vendor/ZF2. Now copy ZF2/* into <ZF2Skeleton folder>/vendor/ZF2 And the final directory structure may look like following image (for ZF2 version 2.3.1 dated 20140426)
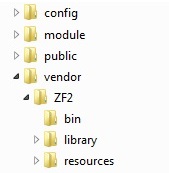
4. Now point any domain into “public” folder. e.g. zf2.localhost.tld
- 4.a. Open notepad as administrator user
- 4.b. Add an entry to your hosts (file) like – “127.0.0.1 zf2.localhost.tld” [one host in each line]
- 4.b.1 hosts file is typically located at C:\WINDOWS\system32\drivers\etc\hosts
- 4.c Now save the hosts file and close
- 4.d. Now create an entry in your IIS (6 or 7 both are same procedure) by following http://support.microsoft.com/kb/816576 with the above host name i.e. zf2.localhost.tld
5. Now you need to fix ZF2_PATH or $zf2Path variable at “/init_autoloader.php” file of root to point our “/vendor/ZF2” folder
Find following code:
$zf2Path = false;
if (getenv(‘ZF2_PATH’)) { // Support for ZF2_PATH environment variable
$zf2Path = getenv(‘ZF2_PATH’);
} elseif (get_cfg_var(‘zf2_path’)) { // Support for zf2_path directive value
$zf2Path = get_cfg_var(‘zf2_path’);
}
Replace by following code:
define(‘DS’, DIRECTORY_SEPARATOR);
define(‘APP_ROOT_PATH’, dirname(__FILE__).DS);
$zf2Path = false;
if (is_dir(APP_ROOT_PATH.’vendor’.DS.’ZF2′.DS.’library’)) {
$zf2Path = APP_ROOT_PATH.’vendor’.DS.’ZF2′.DS.’library’;
} elseif (getenv(‘ZF2_PATH’)) { // Support for ZF2_PATH environment variable or git submodule
$zf2Path = getenv(‘ZF2_PATH’);
} elseif (get_cfg_var(‘zf2_path’)) { // Support for zf2_path directive value
$zf2Path = get_cfg_var(‘zf2_path’);
}
You can now visit zf2.localhost.tld to get your expected site.
Please note, you must run latest copy of PHP (at least bigger then 5.3.23 when I write this article there I found version 5.3.28 stable for windows non thread safe version installer for download) from http://windows.php.net/download/
Now the problem is URL route in IIS. That means when you lookup into ZF2 getting started docs you may find some code to edit .htaccess of apache. What about IIS in this case?
IIS have solution of URL rewrite problem. Visit www.iis.net/urlrewrite to get the latest copy of the plugin to attach into your IIS installation. Just install this addon/plugin/extension/demon/program whatever name you called.
After installation you need a file web.config into your “<ZF2Skeleton folder>/public” folder. No matter, here we do a small trick. Just download Drupal 7+ core from https://drupal.org/project/drupal and unpack it. There you found a web.config file at root path. Just copy and paste that file into your <ZF2Skeleton folder>/public folder.
That’s all folk!